Home › Forums › GetFund – A Professional Laravel Crowdfunding Platform › Bank Account Number › Reply To: Bank Account Number
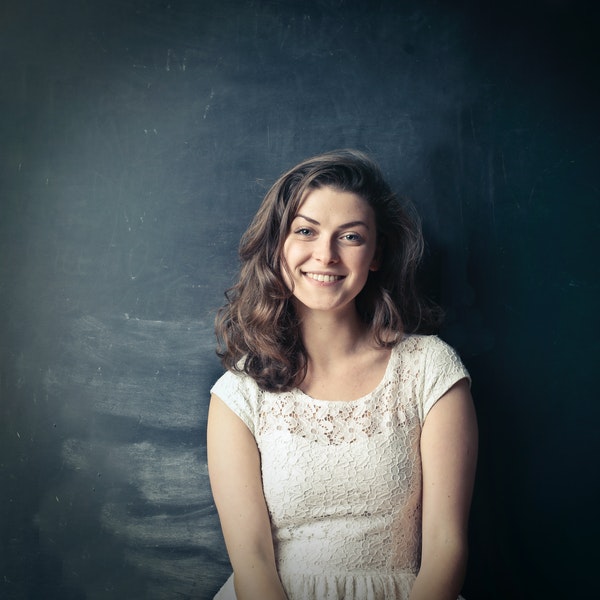
Katherine J. Bates
Hi @kezzlock
Please find the ChangeLog below.
1. Added a database called bank_payments
, Please import it into your current database. below Migration.
Migration File name: 2018_06_03_183905_create_bank_payments.php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateBankPayments extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('bank_payments', function (Blueprint $table) {
$table->increments('id');
$table->integer('user_id')->nullable();
$table->integer('campaign_id')->nullable();
$table->string('bank_swift_code')->nullable();
$table->string('account_number')->nullable();
$table->string('branch_name')->nullable();
$table->string('branch_address')->nullable();
$table->string('account_name')->nullable();
$table->string('iban')->nullable();
$table->text('notes')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('bank_payments');
}
}
2. Routes Lists, add below route to web.php
line number 171 under group > admin > users
Route::get('add-bank/{id}', ['as'=>'add_users_bank_account', 'uses' => 'UserController@addBankAccount']);
Route::post('add-bank/{id}', 'UserController@addBankAccountPost');
Route::get('bank-edit/{id}', ['as'=>'bank_edit', 'uses' => 'UserController@bankEdit']);
Route::post('bank-edit/{id}', 'UserController@bankEditPost');
Route::get('bank-delete/{id}', ['as'=>'bank_delete', 'uses' => 'UserController@bankDelete']);
3. Model > Add BankPayment.php
from app directory
4. Controller, Add below methods to UserController
public function addBankAccount($id){
if ($id){
$title = trans('app.add_bank');
$user = User::find($id);
$campaigns = Campaign::active()->orderBy('created_at', 'desc')->get();
return view('admin.add_bank', compact('title', 'user', 'campaigns'));
}
}
public function addBankAccountPost(Request $request, $id){
$rules = [
'campaign_id' => 'required',
'bank_swift_code' => 'required',
'account_number' => 'required',
'branch_name' => 'required',
'branch_address' => 'required',
'account_name' => 'required',
];
$this->validate($request, $rules);
$check_duplicate = BankPayment::whereUserId($id)->whereCampaignId($request->campaign_id)->first();
if ($check_duplicate){
return back()->with('error', trans('app.bank_payment_exists_info'));
}
$bank_data = array_except($request->input(), '_token');
$bank_data['user_id'] = $id;
BankPayment::create($bank_data);
return back()->with('success', trans('app.bank_payment_created'));
}
public function bankEdit($id){
$bank = BankPayment::find($id);
if ($bank){
$title = trans('app.edit_bank');
$campaigns = Campaign::active()->orderBy('created_at', 'desc')->get();
return view('admin.edit_bank', compact('title', 'bank', 'campaigns'));
}
}
public function bankEditPost(Request $request, $id){
$bank = BankPayment::find($id);
$rules = [
'bank_swift_code' => 'required',
'account_number' => 'required',
'branch_name' => 'required',
'branch_address' => 'required',
'account_name' => 'required',
];
$this->validate($request, $rules);
$bank_data = array_except($request->input(), '_token');
$bank->update($bank_data);
return redirect(route('add_users_bank_account', $bank->user_id))->with('success', trans('app.bank_payment_updated'));
}
public function bankDelete($id){
BankPayment::find($id)->delete();
return back()->with('success', trans('app.bank_payment_deleted'));
}
Replace the checkoutPost
fromCampaignsController
with below method
public function checkoutPost(Request $request){
$title = trans('app.checkout');
if ( ! session('cart')){
return view('checkout_empty', compact('title'));
}
$cart = session('cart');
$input = array_except($request->input(), '_token');
session(['cart' => array_merge($cart, $input)]);
if(session('cart.cart_type') == 'reward'){
$reward = Reward::find(session('cart.reward_id'));
$campaign = Campaign::find($reward->campaign_id);
}elseif (session('cart.cart_type') == 'donation'){
$campaign = Campaign::find(session('cart.campaign_id'));
}
$bank = false;
if (Auth::check()){
$user_id = Auth::user()->id;
$bank = BankPayment::whereUserId($user_id)->whereCampaignId($campaign->id)->first();
}
//dd(session('cart'));
return view('payment', compact('title', 'campaign', 'bank'));
}
6. Views, Copy two files from existing directory to your directory
/root/resources/views/admin/add_bank.blade.php
/root/resources/views/admin/edit_campaign.blade.php
Replace the below file
/root/resources/views/payment.blade.php
7. Language Strings, Add the below array items to
/root/resources/lang/en/app.php
/**
* Customization for Michal
*/
'min_amount_instruction' => 'Minimum amount must be at least',
'add_bank' => 'Add Bank',
'select_campaign' => 'Select Campaign',
'notes' => 'Notes',
'bank_payment_created' => 'Bank Payment info has been created',
'bank_payment_updated' => 'Bank Payment info has been Updated',
'bank_payment_deleted' => 'Bank Payment info has been Deleted',
'bank_payment_exists_info' => 'A bank payment method already exists with selected campaign and this user',
'edit_bank' => 'Edit Bank',
<h4>Min Amount Restrictions</h4>
Replace below two files to add min amount field
/root/resources/views/admin/start_campaign.blade.php
/root/resources/views/admin/edit_campaign.blade.php
Add below code line to /root/resources/views/layouts/footer.blade.php
right after @yield('page-js')
@yield('campaign-sidebar-js')
Replace the file /root/resources/views/campaign_single_sidebar.blade.php
This the complete change log, Please let me know if you facing any issue.
Best Regards